%%raw
![]() |
Home | Web Programming Basics | JS_Overview | Collision | Reflection |
function checkForOverlap(object1,object2){
var pos1 = object1.ReturnPosition().slice();
var scale1 = object1.ReturnScale().slice();
var xRange1 = [pos1[0],pos1[0]+scale1[0]];
var yRange1 = [pos1[1],pos1[1]+scale1[1]];
var pos2 = object2.ReturnPosition().slice();
var scale2 = object2.ReturnScale().slice();
var xRange2 = [pos2[0],pos2[0]+scale2[0]];
var yRange2 = [pos2[1],pos2[1]+scale2[1]];
if (xRange1[0]>=xRange2[0]){
if (xRange1[0]<=xRange2[1]){
if (yRange1[0]>=yRange2[0]){
if (yRange1[0]<=yRange2[1]){
return true;
}
}
if (yRange1[1]>=yRange2[0]){
if (yRange1[1]<=yRange2[1]){
return true;
}
}
}
}
if (xRange1[1]>=xRange2[0]){
if (xRange1[1]<=xRange2[1]){
if (yRange1[0]>=yRange2[0]){
if (yRange1[0]<=yRange2[1]){
return true;
}
}
if (yRange1[1]>=yRange2[0]){
if (yRange1[1]<=yRange2[1]){
return true;
}
}
}
}
return false;
}
Problem with this code
- This code uses too many if statements, which causes it be way too complex and hard to follow.
Revised code
function checkForOverlap(object1, object2) {
var pos1 = object1.ReturnPosition();
var scale1 = object1.ReturnScale();
var xRange1 = [pos1[0], pos1[0] + scale1[0]];
var yRange1 = [pos1[1], pos1[1] + scale1[1]];
var pos2 = object2.ReturnPosition();
var scale2 = object2.ReturnScale();
var xRange2 = [pos2[0], pos2[0] + scale2[0]];
var yRange2 = [pos2[1], pos2[1] + scale2[1]];
function rangesOverlap(range1, range2) {
return range1[0] < range2[1] && range1[1] > range2[0];
}
return (
rangesOverlap(xRange1, xRange2) && rangesOverlap(yRange1, yRange2)
);
}
Revised Code explanation
1.
var pos1 = object1.ReturnPosition();
pos1: This variable stores the position (coordinates) of the first object (object1). object1.ReturnPosition(): Calls a method (ReturnPosition()) that provides the position of object1.
-
var scale1 = object1.ReturnScale();
scale1: This variable stores the size or scale (width and height) of object1. object1.ReturnScale(): Calls a method (ReturnScale()) that gives the scale information of object1.
3.
var xRange1 = [pos1[0], pos1[0] + scale1[0]];
xRange1: This is an array representing the range along the x-axis for object1. pos1[0]: The starting point of the object on the x-axis. pos1[0] + scale1[0]: The ending point of the object on the x-axis (start + width).
4.
var yRange1 = [pos1[1], pos1[1] + scale1[1]];
yRange1: This array represents the range along the y-axis for object1. pos1[1]: The starting point of the object on the y-axis. pos1[1] + scale1[1]: The ending point of the object on the y-axis (start + height).
5.
function rangesOverlap(range1, range2)
rangesOverlap: This is a function that checks if two ranges overlap. range1 and range2 are arrays representing ranges along an axis.
6.
return range1[0] < range2[1] && range1[1] > range2[0];
return: This line returns true or false based on the condition. This condition checks if the start of one range is less than the end of the other range, and also if the end of the first range is greater than the start of the other range along an axis.
7.
return (rangesOverlap(xRange1, xRange2) && rangesOverlap(yRange1, yRange2));
Final return statement: It uses the rangesOverlap function to check if the x-axis ranges and y-axis ranges of two objects overlap. It returns true if both the x-axis and y-axis ranges overlap for both objects.
Diagram to explain better
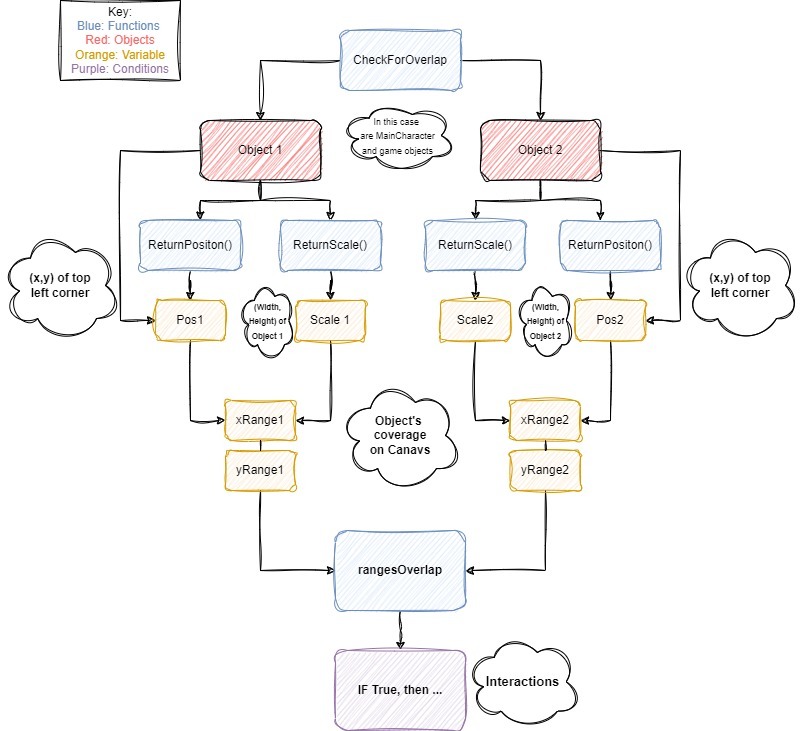
Overlap code implementation
if (checkForOverlap(myCharacterObject, doorObject)) {
console.log("Now press the E key");
}
Code Explanation
This code takes the two objects (character and box) from The original code (Ctrl+Shift+I -> console), that were defined earlier.
- Takes the overlap code, and if presents as True, message is played
- Now when the checkForOverlap sees the two objects of the Character and Object ovlerap, (Using the Overlap Code) it pastes the message “Now Press the P key” in the console. as seen in the following:
- Now that I know the collision code works, I went on to add the sprite and interaction with the boxes.
Ekey
First it creates an object called ekey Interaction with Ekey: There is an event listener added to the window for the ‘keydown’ event. When the ‘E’ key is pressed (key code 69), it checks for the overlap between the main character and a BoxObject1 (Using Overlap Code). If an overlap is detected, it alters the scale of the boxes, making it disappear.
Condition for Displaying the Ekey Sprite
When the main character overlaps with the boxes, a variable named showEKeySprite is set to true. Frame Update Function:
- If this is true, Ekey sprite animation is updated.
- The Ekey sprite is drawn on the canvas using Ekey.draw()
- Within the frame() function, the code updates the Ekey sprite animation at a set frame rate using the condition currentFrame % Math.round(fps/2)==0.
- This calculation is used to control the frequency of sprite updates, giving the appearance of animation.
## Drawings I made used in game
Drawings I made not used in game
Resourcse I used:
Drawings I made used in game
Drawings I made not used in game